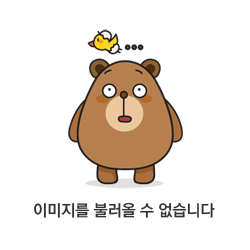
자바빈즈부터 시작..
자바가 서버쪽에서 각광을 받고,
화면에 안보여지는 쪽에서 Bean을 쓰게 됨
MVC에서 모델 역할을 하고(데이터 전달할 때), EL, Scope에서 쓰임.. - JSP container가 관리
EJB가 나옴 -> EJP Container가 관리
관리란? 빈을 생성하고 소멸시키는 것
Spring Bean --> EJB의 복잡한 규칙을 단순하게 만든 것, 단순/독립적 - Spring Container가 관리
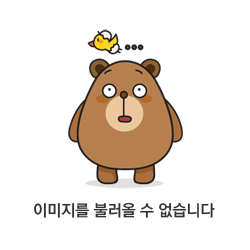
1. 스프링 컨테이너(Spring Container)
스프링에서 의존관계 주입(Dependency Injection, DI)을 이용하여 애플리케이션을 구성하는 여러 빈(Bean)들의 생명주기(Lifecycle)와 애플리케이션의 서비스 실행 등을 관리하며 생성된 인스턴스들에게 기능을 제공하는 것을 부르는 말이다.
컨테이너에 적절한 설정만 있다면 프로그래머의 개입 없이도 작성된 코드를 컨테이너가 빈을 스스로 참조한 뒤 알아서 관리한다.
스프링 컨테이너를 언급할때는 빈 팩토리(BeanFactory)와 애플리케이션 컨텍스트(ApplicationContext) 두 가지로 다룬다.
스프링 컨테이너가 빈을 생성/소멸/연결(@Autowired)
BeanFactory는 인터페이스 --> 빈 생성, 연결
그것을 확장한 것이 AppicationContext --> 생성,연결 뿐만 아니라 다른 추가기능을 가지고 있음
(Application context의 일부가 Spring Container)
https://beststar-1.tistory.com/39
스프링 컨테이너(BeanFactory, ApplicationContext)
스프링 컨테이너(Spring Container) 스프링에서 의존관계 주입(Dependency Injection, DI)을 이용하여 애플리케이션을 구성하는 여러 빈(Bean)들의 생명주기(Lifecycle)와 애플리케이션의 서비스 실행 등을 관리
beststar-1.tistory.com
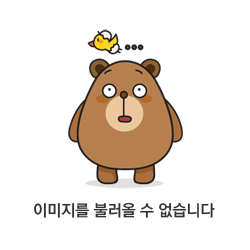
ApplicationContext 또한 인터페이스다
설정을 XML / JAVA 코드 둘 중 하나로 할 수 있다
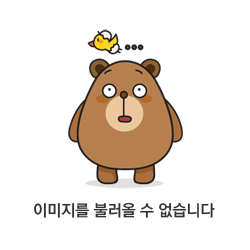
Spring MVC는 web, xml을 사용하려면 xmlwebapplication을 사용해야한다
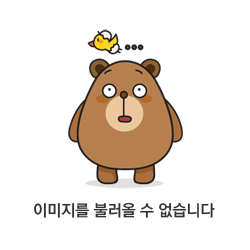
톰캣 엔진 안에
호스트별로 영역이 있고,
모듈별로 컨텍스트가 있다
그 안에 서블릿들이 있는데,
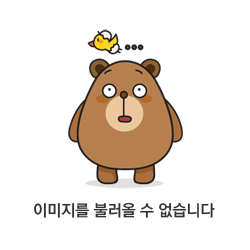
ApplicationContext는 JSP의 기본 객체 application
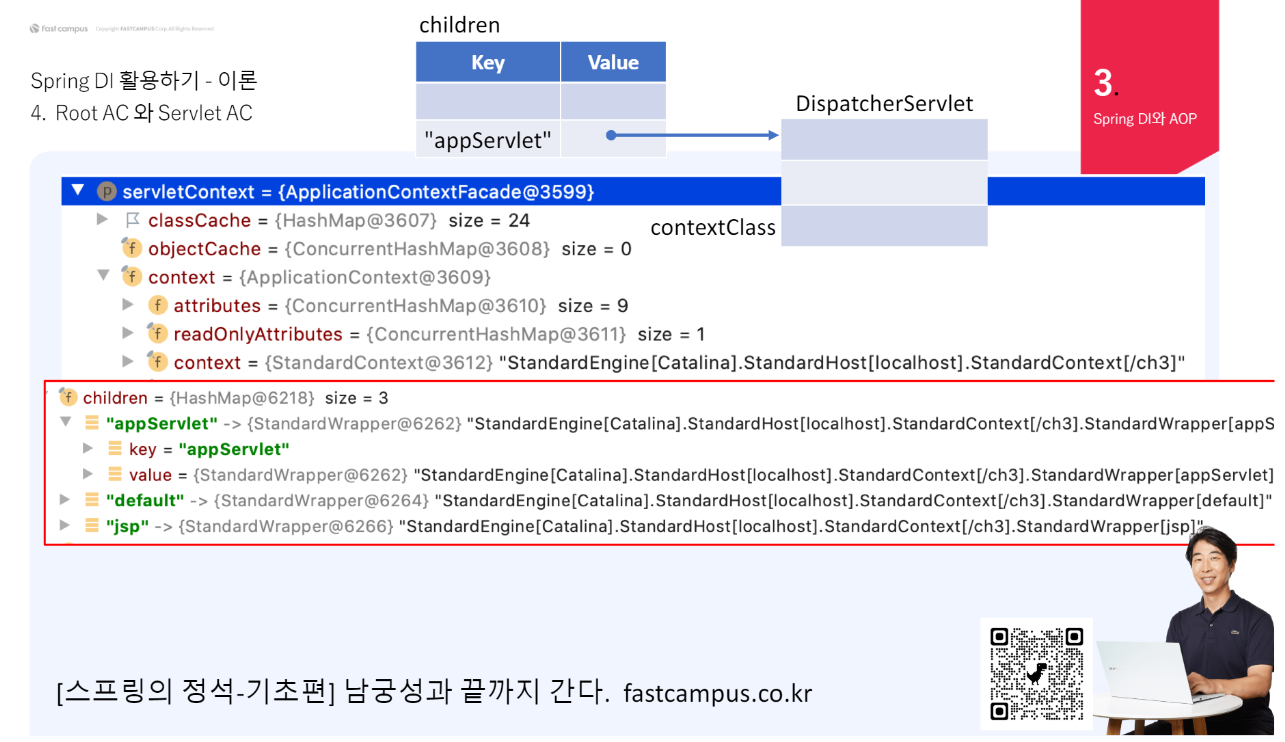
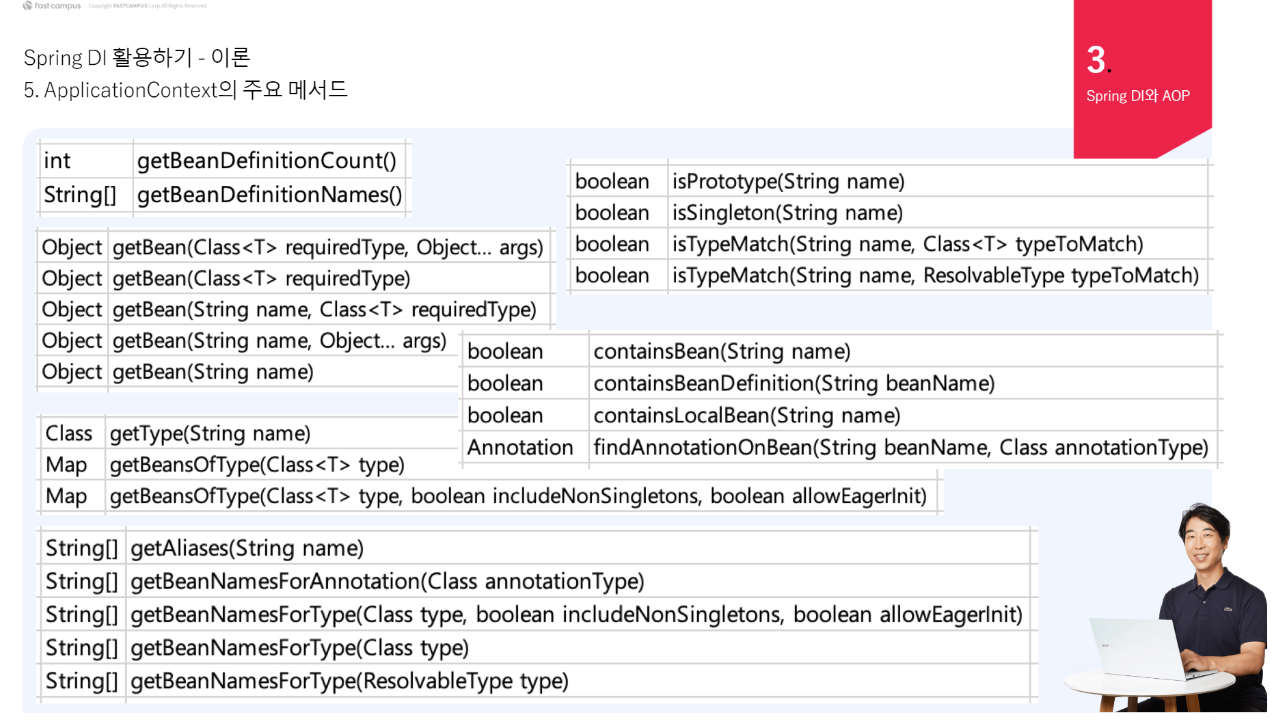
자주 쓸만한 주요 메서드들..
<java />
package com.fastcampus.ch3;
import org.springframework.beans.factory.annotation.*;
import org.springframework.context.*;
import org.springframework.context.annotation.*;
import org.springframework.context.annotation.Scope;
import org.springframework.context.support.*;
import org.springframework.stereotype.*;
import javax.inject.*;
import java.util.*;
@Component
@Scope("prototype")
class Door {}
@Component class Engine {}
@Component class TurboEngine extends Engine {}
@Component class SuperEngine extends Engine {}
@Component
class Car {
@Value("red") String color;
@Value("100") int oil;
@Autowired Engine engine;
@Autowired Door[] doors;
@Override
public String toString() {
return "Car{" +
"color='" + color + '\'' +
", oil=" + oil +
", engine=" + engine +
", doors=" + Arrays.toString(doors) +
'}';
}
}
public class ApplicationContextTest {
public static void main(String[] args) {
ApplicationContext ac = new GenericXmlApplicationContext("config.xml");
// Car car = ac.getBean("car", Car.class); // 타입을 지정하면 형변환 안해도됨. 아래의 문장과 동일
Car car = (Car) ac.getBean("car"); // 이름으로 빈 검색
Car car2 = (Car) ac.getBean(Car.class); // 타입으로 빈 검색
System.out.println("car = " + car);
System.out.println("car2 = " + car2);
System.out.println("ac.getBeanDefinitionNames() = " + Arrays.toString(ac.getBeanDefinitionNames())); // 정의된 빈의 이름을 배열로 반환
System.out.println("ac.getBeanDefinitionCount() = " + ac.getBeanDefinitionCount()); // 정의된 빈의 개수를 반환
System.out.println("ac.containsBeanDefinition(\"car\") = " + ac.containsBeanDefinition("car")); // true 빈의 정의가 포함되어 있는지 확인
System.out.println("ac.containsBean(\"car\") = " + ac.containsBean("car")); // true 빈이 포함되어 있는지 확인
System.out.println("ac.getType(\"car\") = " + ac.getType("car")); // 빈의 이름으로 타입을 알아낼 수 있음.
System.out.println("ac.isSingleton(\"car\") = " + ac.isSingleton("car")); // true 빈이 싱글톤인지 확인
System.out.println("ac.isPrototype(\"car\") = " + ac.isPrototype("car")); // false 빈이 프로토타입인지 확인
System.out.println("ac.isPrototype(\"door\") = " + ac.isPrototype("door")); // true
System.out.println("ac.isTypeMatch(\"car\", Car.class) = " + ac.isTypeMatch("car", Car.class)); // "car"라는 이름의 빈의 타입이 Car인지 확인
System.out.println("ac.findAnnotationOnBean(\"car\", Component.class) = " + ac.findAnnotationOnBean("car", Component.class)); // 빈 car에 @Component가 붙어있으면 반환
System.out.println("ac.getBeanNamesForAnnotation(Component.class) = " + Arrays.toString(ac.getBeanNamesForAnnotation(Component.class))); // @Component가 붙은 빈의 이름을 배열로 반환
System.out.println("ac.getBeanNamesForType(Engine.class) = " + Arrays.toString(ac.getBeanNamesForType(Engine.class))); // Engine 또는 그 자손 타입인 빈의 이름을 배열로 반환
}
}
'패캠 챌린지' 카테고리의 다른 글
Spring으로 DB 연결하기 (0) | 2023.03.28 |
---|---|
스프링 IOC와 DI (0) | 2023.03.24 |
스프링 DI 활용하기 - 실습 (0) | 2023.03.22 |
스프링 DI 흉내내기 - 객체 찾는 법 (by type/by name) (0) | 2023.03.22 |
패스트캠퍼스 챌린지 - 30일차 [스프링의 정석:남궁성과 끝까지 간다] (0) | 2023.03.21 |